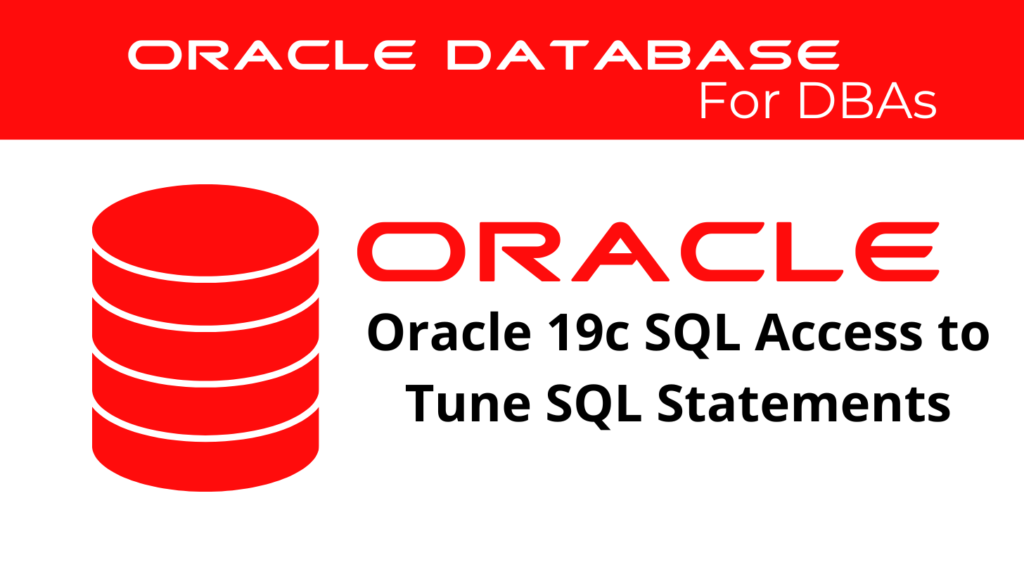
Optimizing SQL performance is crucial for maintaining an efficient database system. In Oracle 19c, SQL Access helps DBAs to tune SQL statements, leading to significant improvements in database operations. By utilizing SQL Access, one can effectively manage and optimize SQL statements, resulting in enhanced performance and resource utilization. This tutorial will guide you through the processes and best practices to tune SQL statements using SQL Access in Oracle 19c, focusing on SQL Access Advisor.
Understanding SQL Access
SQL Access refers to various methods and tools used to analyze and optimize SQL queries. In Oracle 19c, it encompasses the use of SQLAccess Advisor, which provides recommendations for improving query performance through indexing, partitioning, and materialized views. Understanding the SQLAccess methodologies and tools is essential for any DBA aiming to enhance database performance.
The Role of SQL Access Advisor
SQL Access Advisor is a key component in SQL Tune. It helps DBAs make informed decisions on creating, retaining, or dropping indexes, materialized views, and partitions. This advisor accepts input from multiple sources and simplifies the tuning process, making it accessible even for those without deep expertise in SQL optimization. By utilizing the SQLAccess Advisor, you can significantly improve the efficiency of SQL queries and overall database performance.
Implementing SQL Access Advisor Recommendations
To use SQL Access Advisor effectively, one must follow a structured approach. Here’s how you can implement its recommendations:
Creating SQL Tuning Sets
SQL Tuning Sets (STS) are essential for storing SQL statements along with their execution context. These sets serve as input for SQL Access Advisor. Creating an STS involves defining the set and loading it with relevant SQL statements from your workload.
EXEC DBMS_SQLTUNE.CREATE_SQLSET(sqlset_name => 'my_sql_tuning_set', description => 'Tuning set for sales queries');
Loading SQL Tuning Sets
Populate the STS with SQL statements from your workload. This step ensures that the SQL Access Advisor has accurate and comprehensive data for analysis.
DECLARE
sqlset_cursor DBMS_SQLTUNE.SQLSET_CURSOR;
BEGIN
OPEN sqlset_cursor FOR
SELECT VALUE(t) FROM TABLE (DBMS_SQLTUNE.SELECT_CURSOR('SELECT * FROM sales')) t;
DBMS_SQLTUNE.LOAD_SQLSET('my_sql_tuning_set', sqlset_cursor);
END;
Executing SQL Access Advisor Tasks
Define and execute tasks to generate recommendations. This process involves creating a task, setting its parameters, linking it to an STS, and executing it.
DECLARE
task_id NUMBER;
task_name VARCHAR2(255);
BEGIN
task_name := 'my_sql_optimization_task';
DBMS_ADVISOR.CREATE_TASK('SQL Access Advisor', task_id, task_name);
DBMS_ADVISOR.SET_TASK_PARAMETER(task_name, 'ANALYSIS_SCOPE', 'ALL');
DBMS_ADVISOR.ADD_STS_REF(task_name, 'SH', 'my_sql_tuning_set');
DBMS_ADVISOR.EXECUTE_TASK(task_name);
END;
Viewing Recommendations
Recommendations can be viewed through SQL queries or graphical interfaces. This step allows you to review the suggested actions and implement them to improve SQL performance.
SELECT rec_id, rank, benefit FROM USER_ADVISOR_RECOMMENDATIONS WHERE task_name = 'my_sql_optimization_task' ORDER BY rank;
Indexing Strategies
Indexes are crucial for speeding up query execution. SQL Access Advisor can recommend various types of indexes, such as B-tree, bitmap, and function-based indexes. Understanding and implementing the right indexing strategy is vital for optimizing query performance.
Creating and Dropping Indexes
Creating indexes involves specifying the columns to be indexed. Dropping unnecessary indexes can also enhance performance by reducing overhead.
CREATE INDEX idx_customer_name ON customers (last_name, first_name);
DROP INDEX idx_customer_name;
📢 You might also like: Oracle 19c SQL Tuning Advisors to Tune SQL Statements (Category: Performance Management and Tuning)
Utilizing Materialized Views
Materialized views store the results of a query, allowing for faster data retrieval. SQL Access Advisor provides recommendations on creating and optimizing materialized views to enhance performance. Proper use of materialized views can significantly reduce query execution time.
Creating and Dropping Materialized Views
Creating materialized views involves defining the query results to be stored. Dropping outdated or unused materialized views helps maintain database efficiency.
CREATE MATERIALIZED VIEW sales_mv
AS SELECT time_id, prod_id, SUM(amount_sold) AS total_sales
FROM sales
GROUP BY time_id, prod_id;
DROP MATERIALIZED VIEW sales_mv;
Effective Partitioning
Partitioning divides large tables into smaller, more manageable pieces, improving performance and manageability. SQL Access Advisor can suggest partitioning strategies based on the workload. Effective partitioning strategies can greatly enhance query performance and simplify data management.
Creating Partitioned Tables
Creating partitioned tables involves defining the partitioning criteria and creating the partitions. This helps in distributing data efficiently across different storage segments.
CREATE TABLE sales_partitioned
(time_id DATE, prod_id NUMBER, amount_sold NUMBER)
PARTITION BY RANGE (time_id)
(PARTITION p1 VALUES LESS THAN (TO_DATE('2020-01-01', 'YYYY-MM-DD')),
PARTITION p2 VALUES LESS THAN (TO_DATE('2021-01-01', 'YYYY-MM-DD')));
Gathering Statistics
Accurate statistics are essential for the optimizer to make informed decisions. Use the DBMS_STATS package to gather these statistics. Keeping statistics up-to-date ensures that the optimizer has the necessary information to generate optimal execution plans.
Gathering Table and Index Statistics
Gathering statistics involves analyzing tables and indexes to collect information about data distribution and storage. This data helps the optimizer make better decisions.
EXEC DBMS_STATS.GATHER_TABLE_STATS('SH', 'SALES');
EXEC DBMS_STATS.GATHER_INDEX_STATS('SH', 'IDX_SALES_TIME');
Advanced SQL Tuning
Advanced tasks include evaluating existing access structures and updating task attributes to refine the tuning process. These tasks require a deeper understanding of SQL Access and the database environment.
Evaluating Existing Access Structures
Evaluation mode allows you to review and assess the effectiveness of current access structures without making changes.
EXEC DBMS_ADVISOR.SET_TASK_PARAMETER('mytask', 'ANALYSIS_SCOPE', 'EVALUATION');
Updating Task Attributes
Updating task attributes involves modifying parameters to better align the tuning process with specific requirements.
EXEC DBMS_ADVISOR.UPDATE_TASK_ATTRIBUTES('mytask', 'TUNING1');
EXEC DBMS_ADVISOR.UPDATE_TASK_ATTRIBUTES('TUNING1', read_only => 'true');
SQL Access Advisor in Action
To illustrate the use of SQL Access Advisor, consider a scenario where a DBA needs to optimize a complex query workload for a sales database. By following the steps outlined, the DBA can create and load SQL Tuning Sets, execute tasks, and implement the recommendations provided by SQL Access Advisor.
Creating the SQL Tuning Set
First, the DBA creates a SQL Tuning Set named sales_sql_tuning_set
to store the relevant SQL statements.
EXEC DBMS_SQLTUNE.CREATE_SQLSET(sqlset_name => 'sales_sql_tuning_set', description => 'Tuning set for sales queries');
Loading the SQL Tuning Set
Next, the DBA populates the tuning set with SQL statements that are frequently used in the sales database.
DECLARE
sqlset_cursor DBMS_SQLTUNE.SQLSET_CURSOR;
BEGIN
OPEN sqlset_cursor FOR
SELECT VALUE(t) FROM TABLE (DBMS_SQLTUNE.SELECT_CURSOR('SELECT * FROM sales WHERE sales_date BETWEEN TO_DATE(''2023-01-01'', ''YYYY-MM-DD'') AND TO_DATE(''2023-12-31'', ''YYYY-MM-DD'')')) t;
DBMS_SQLTUNE.LOAD_SQLSET('sales_sql_tuning_set', sqlset_cursor);
END;
Executing the SQL Access Advisor Task
The DBA then creates and executes a task to analyze the SQL statements and generate optimization recommendations.
DECLARE
task_id NUMBER;
task_name VARCHAR2(255);
BEGIN
task_name := 'sales_optimization_task';
DBMS_ADVISOR.CREATE_TASK('SQL Access Advisor', task_id, task_name);
DBMS_ADVISOR.SET_TASK_PARAMETER(task_name, 'ANALYSIS_SCOPE', 'ALL');
DBMS_ADVISOR.ADD_STS_REF(task_name, 'SH', 'sales_sql_tuning_set');
DBMS_ADVISOR.EXECUTE_TASK(task_name);
END;
Reviewing and Implementing Recommendations
Finally, the DBA reviews the recommendations and implements them to enhance the performance of the sales database queries.
SELECT rec_id, rank, benefit FROM USER_ADVISOR_RECOMMENDATIONS WHERE task_name = 'sales_optimization_task' ORDER BY rank;
BEGIN
FOR rec IN (SELECT * FROM USER_ADVISOR_RECOMMENDATIONS WHERE task_name = 'sales_optimization_task')
LOOP
DBMS_OUTPUT.PUT_LINE('Recommendation ID: ' || rec.rec_id || ' Benefit: ' || rec.benefit);
END LOOP;
END;
Conclusion
Optimizing SQL statements in Oracle 19c using SQLAccess and SQL Access Advisor is essential for maintaining high-performance databases. By following the recommendations and implementing the suggested changes, DBAs can ensure that their databases operate efficiently and effectively. SQL Access to tune SQL statements is a powerful approach that, when utilized correctly, can lead to substantial performance improvements.
See more on Oracle’s website!
Be Oracle Performance Management and Tuning Certified Professional, this world is full of opportunities for qualified DBAs!